I know we have a couple Python programmers here. I've been working on a little script designed to make it easier for new programmers who want to make games to just get started on the game logic, rather than all the potentially daunting graphical stuff. It's also just fun to play with if you're already experienced; I mostly made it because I wanted it to exist.
It allows you to create a grid and customize it's size, shape, colour, etc. and control individual cells to make grid based games.
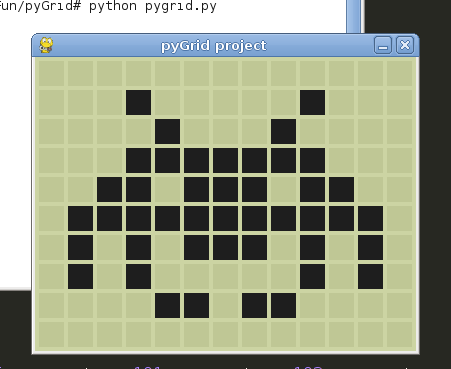
I could probably tell you best through code snippets:
# There are two ways to instantiate the grid
# You can include all arguments immediately upon instantiation
# (grid_width,grid_height,cell_width,cell_height,border_weight,border_color,off_color,on_color,radius,title)
grid = pygrid.pyGrid(10, 10, 15, 15, 3, (0,0,0), (50,50,50), (0,255,0), 4, "Window Title")
# ...or you can set all attributes individually, which is easier initially
grid = pygrid.pyGrid()
# set number of horizontal and vertical cells
grid.width = 10
grid.height = 10
# set width and height of cells in pixels
grid.cell_width = 15
grid.cell_height = 15
# set size/color of margins/borders
grid.border_weight = 3
grid.border_color = (0,0,0)
# set default off/on colours of cells
grid.off_color = (50,50,50)
grid.on_color = (0,255,0)
# set rounded corner radius if desired
grid.radius = 4
# Title
grid.caption = "Window Title"
Once the grid is instantiated (all of the attributes are optional by the way, and if not set will default to the settings of the Space Invader example), using the grid is as simple as turning cells 'on' or 'off.'
grid.on(4,5) #xy coordinate
grid.off(4,5)
# If you want 'on' cells of multiple colours, like the Snake example below,
# you will have to supply 2 further arguments, radius, and colour
grid.on(4,5,0,(0,0,0))
grid.off(4,5)
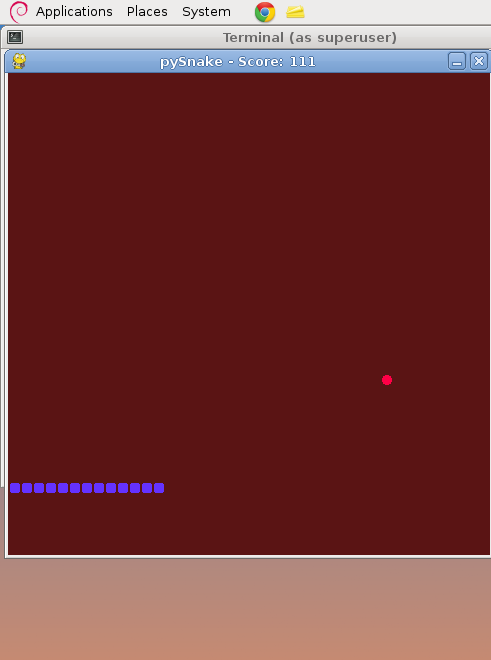
If you want to try it out, you will need Python (<3.0) and PyGame installed. Included is an extremely messy, but functional game of Snake, which should show you how to use it, although it should be simple enough to just figure out on your own.
Currently, I consider it to be at a sort of working prototype stage. I want to clean up the source code (a lot), and change the hackish "list of lists" method of handling the grid coordinates to a "list of x,y tuples" method, which shouldn't be hard but maybe when my lady goes home so I don't bore her to death. That's how I handled the coordinates in the Snake example so I want to quickly adapt it to the pyGrid class. It seems like a far more efficient method, and most of pyGrid was just hacked together to get a prototype going. Keep in mind that I've only been using Python for about a week.
I have a few ideas for other features, but I want to weed out the unnecessary bloat and only put in ones I can see being useful. I was thinking of some functions to make handling the game loop and timing (clock.tick(num)) easier for new programmers, but that's not really hard once you understand it. I was also thinking of some simple drawing functions like grid.draw_line(2,4,6,8), grid.draw_oval(2,4,6,8) but I think those would be fun to code, but in most cases, useless. One feature I am definitely going to include is an image feature, rather than supplying just a colour, one can define a background image for a cell. I'm not sure if it will just be a background image or if I'll allow images larger than the cell, simply using the cell as a point of origin.
And yes, of course I'm coding a Tetris clone.